What is Django:
Django is python framework for development of web applications. This is a opensource framework used for web development. Python has other web development frameworks like Flask, but Django is fully featured web framework compared to light weight Flask.
We are using Ubuntu-20.4 machine as a development host. Django can be installed on Ubuntu using apt-get command.
sudo apt install python3-django
After installation Django version can be verified using
bash# django-admin --version
3.0.3
or we can use pip to install Django in Virtual Environment,
pip install django
Create Hello World Project:
Django project can be created using django-admin startproject <name of the project> as shown below.
bash#django-admin startproject Hello World. Once the project is created using startproject below list of files are created.
bash# tree HelloWorld/
HelloWorld/
├── db.sqlite3
├── HelloWorld
│ ├── asgi.py
│ ├── init.py
│ ├── settings.py
│ ├── urls.py
│ └── wsgi.py
└── manage.py
Django creates two directories with the project name, HelloWorld in this case. The main HelloWorld directory contains manage.py, db.sqlite3 and inner HelloWorld directory.
manage.py: This is main commands scripts used to interact with Django project. To run the project we use python3 manage.p runserver, similarly to create super user manage.py createsuperuser command with manage.py
db.sqlite3 : Django supports sqlite database by default. User can change to their preferred database by giving details in settings.py file. Django create db tables and index for the models created for this project.
HelloWorld/settings.py: settings.py is used to setup various project settings like path of static files, database settings, different middleware (Django python framework) libraries this project depends.
HelloWorld/urls.py: This file contains all the url patterns used in this project. In this url patterns we link Django views and webpages with corresponding url.
HelloWorld/wsgi.py: This script is used for deployment of application to server.
Run Django project:
Django project can be run using the following command, and command output is as shown below.
bash# python3 manage.py runserver
Watching for file changes with StatReloader
Performing system checks…
System check identified no issues (0 silenced).
You have 17 unapplied migration(s). Your project may not work properly until you apply the migrations for app(s): admin, auth, contenttypes, sessions.
Run ‘python manage.py migrate’ to apply them.
November 29, 2020 – 07:48:47
Django version 3.0.3, using settings ‘HelloWorld.settings’
Starting development server at http://127.0.0.1:8000/
Quit the server with CONTROL-C.
Open browser and navigate to http://127.0.0.1:8000/ to open the project. As we not done any changes to the project created by django-admin we see default Django page shown below.
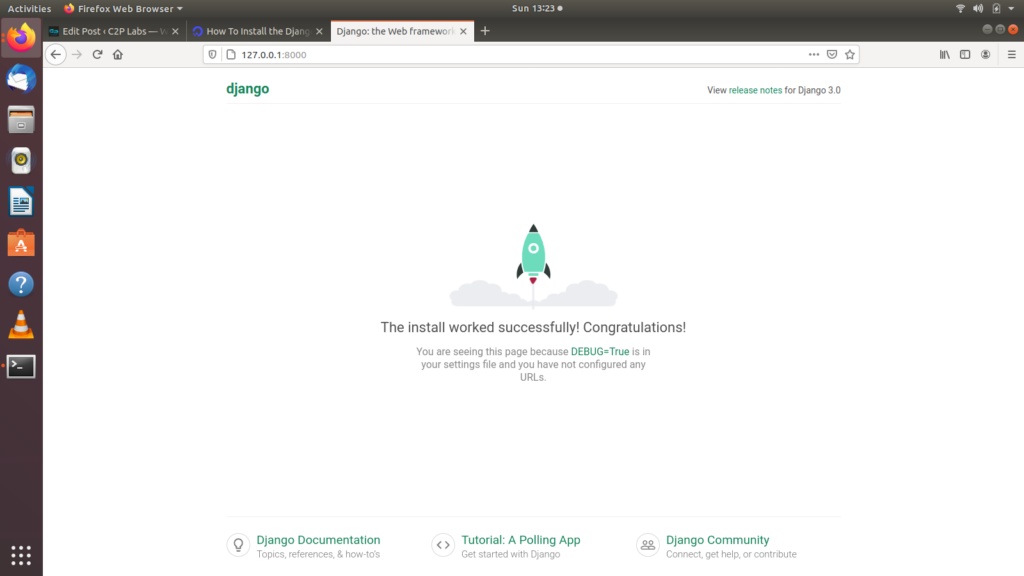
Django Application:
We have seen how to create a Django project. Now we create a Django application. Django project can contain one or more applications. Each Django application is created to perform a dedicated functionality of the large project.
bash# python3 manage.py startapp my_first_app
This command creates the following tree in HelloWorld directory
my_first_app/
├── admin.py
├── apps.py
├── init.py
├── migrations
│ └── init.py
├── models.py
├── tests.py
└── views.py
Among the different files created by Django, we use views.py to create view class and view functions to handle http request from clients.
models.py: The database models created for the application are stored in this file.
Add below lines to my_first_app/views.py
from django.shortcuts import render
from django.http import HttpResponse
# Create your views here.
def index(request):
return HttpResponse("Django Hello World Application !")
~
Add below lines to HelloWorld/urls.py
from django.contrib import admin
from django.urls import path
from my_first_app import views
urlpatterns = [
path('',views.index, name='index'),
path('admin/', admin.site.urls),
]