MQTT is a messaging protocol widely used in the Internet of Things (IoT) to control and access smart devices over the internet. This tutorial demonstrates how to develop a simple MQTT client for the ESP8266 NodeMCU using the Arduino framework. In this demonstration, we utilize the ESP8266 GPIO 12 connected to a relay to control an LED bulb. The ESP8266-12E module comes with an on-board LED connected to GPIO 2. We control these two GPIOs upon receiving messages from an MQTT Publish client. We are using an Android app as the publishing client and the Mosquitto MQTT broker running on a Linux machine.
PubSubClient library is used for developing MQTT subscribe client running on ESP8266.
We develop MQTT subscribe client which subscribes on topic “/root/switch1″
We are using PlatformIO for creating ESP8266 project using Arduino framework. This blog post https://c2plabs.com/blog/2021/12/14/platformio-installation-withudio c-visual-studio-code-and-hello-world-program/ gives details about how to setup PlatformIO in Visual Studio code and create a project.
After creating a project, go to libraries and search for PubSubClient library, install the library and add to the project created as shown below. After adding the library to project platformio.ini file contains “lib_deps = knolleary/PubSubClient@^2.8”
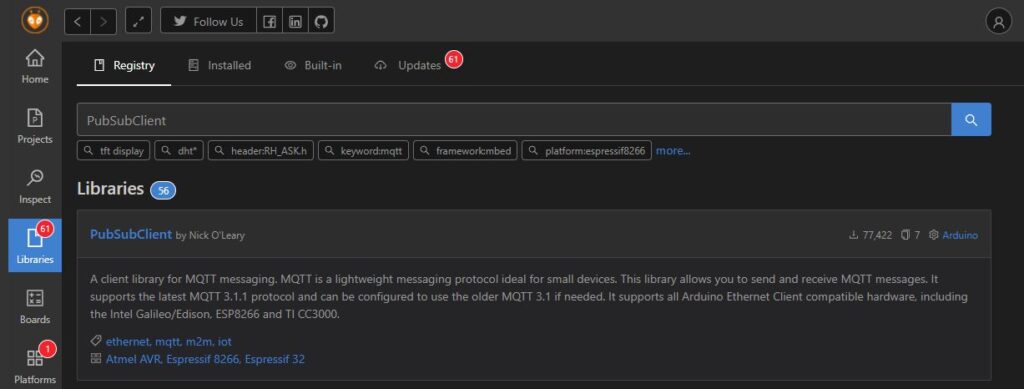
Include the header files required for ESP8266WiFi.h and PubSubClient.h
We are using ESP8266 GPIO 12 for connecting relay and on-board LED connect to GPIO 2.
#define RELAY 12
#define LED 2
Declare char string variables for wifi credentails
const char *ssid=”MYSSID”;
const char *passwd = “MyPasswd”;
We need network client for connecting to Server over TCP protocol. WiFIClient network socket client class which provides socket communication APIs. PubSubClient requires socket client for initializing
WiFiClient wlanclient;
PubSubClient mqttClient(wlanclient);
In setup function we are connecting to WiFi access point with the credentials declared above.
while(WiFi.status()!=WL_CONNECTED) {
Serial.print (“.”);
delay(100);
}
Serial.print (“Connected to WiFi AP, Got an IP address :”);
Serial.print (WiFi.localIP());
Once the WiFi connection established successfully, Setup MQTT broker detail and connect to MQTT broker. setServer method takes arguments like MQTT broker IP address and port. Default port on which MQTT broker listens is 1883.
MQTT Client takes a callback function. This function is called when there is a data on the topic subscribed to, This mqttCallback function needs to be implemented.
mqttClient.setServer (“192.168.1.17”,1883);
mqttClient.setCallback(mqttCallback);
Connect method takes arguments like client name, username and password. We are not using any username and password in our example.
mqttClient.connect (“ESP-Client”,NULL,NULL))
After connecting to MQTT broker, subscribe to the topic /root/switch1, this is the topic used in MQTT Publishing client.
mqttClient.subscribe(“/root/switch1”);
After setup is done, call mqttClient.loop() in main loop function of the ESP266 Arduino.
Implementing MQTT Client (PubSubClient) Callback function:
PubSubClient callback function takes three arguments
Pointer to topic, pointer to mqtt message payload which is a byte array, and length of the message received.
void mqttCallback(char *topic, byte *payload, unsigned int length)
Copy the payload into character string and then to Arduino String class, and validate the message received from Publish client. If the message received is ‘ON‘, Turn on the RELAY and LED by writing active high on GPIOs, if the message received is OFF, turn of the RELAY and LED.
Complete source code is given below.
#include <Arduino.h>
#include <ESP8266WiFi.h>
#include <PubSubClient.h>
#define RELAY 12
#define LED 2
const char *ssid="MYSSID";
const char *passwd = "MyPasswd";
WiFiClient wlanclient;
PubSubClient mqttClient(wlanclient);
void mqttCallback(char *topic, byte *payload, unsigned int length) {
Serial.print ("Message arrived on Topic:");
Serial.print (topic);
char message[5]={0x00};
for(int i=0;i<length;i++)
message[i]=(char)payload[i];
message[length]=0x00;
Serial.print (message);
String str_msg = String(message);
if(str_msg.equals ("ON")) {
digitalWrite(RELAY,HIGH);
digitalWrite(LED,HIGH);
}else if (str_msg.equals("OFF")) {
digitalWrite (RELAY,LOW);
digitalWrite (LED,LOW);
}
}
void setup() {
Serial.begin (115200);
pinMode (RELAY,OUTPUT);
pinMode(LED,OUTPUT);
WiFi.begin(ssid,passwd);
Serial.print ("Connecting to AP");
while(WiFi.status()!=WL_CONNECTED) {
Serial.print (".");
delay(100);
}
Serial.print ("Connected to WiFi AP, Got an IP address :");
Serial.print (WiFi.localIP());
mqttClient.setServer ("192.168.1.17",1883);
mqttClient.setCallback(mqttCallback);
if(mqttClient.connect ("ESP-Client",NULL,NULL)) {
Serial.print ("Connected to MQTT Broker");
} else {
Serial.print("MQTT Broker connection failed");
Serial.print (mqttClient.state());
delay(200);
}
mqttClient.subscribe("/root/switch1");
}
void loop() {
mqttClient.loop();
}